Checkboxes allow the user to select one or more options from a set.
In this tutorial, we show you how to customize a checkbox, add a click listener by a few different ways, and how to get the setOnCheckedChangeListener for checkbox.
<CheckBox
android:id="@+id/checkBox1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="CheckBox 1"
android:onClick="onCheckboxClicked"
android:button="@drawable/checkbox_selector"/>
<CheckBox
android:id="@+id/checkBox2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="CheckBox 2"
android:onClick="onCheckboxClicked"
android:layout_marginBottom="20dp"
android:button="@drawable/checkbox_selector" />
We need a xml file “checkbox_selector” in folder drawable:
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_checked="true"
android:drawable="@drawable/check" />
<item android:state_checked="false"
android:drawable="@drawable/uncheck" />
</selector>
And of course two image with name “check” and “uncheck” for two state of checkbox.
2. Two ways to attach a click listener to the checkbox, when user click on the checkbox, show info on the textview control
2.1. Use android:onClick attribute in your checkbox XML definition:
android:onClick="onCheckboxClicked"
Within the activity that hosts this layout, the following method handles the click event for both checkboxes:
public void onCheckboxClicked(View view) {
// Is the view now checked?
boolean checked = ((CheckBox) view).isChecked();
// Check which checkbox was clicked
switch(view.getId()) {
case R.id.checkBox1:
if (checked)
textview.setText("checkbox 1 is check by onclick from xml");
else
textview.setText("checkbox 1 is uncheck by onclick from xml");
break;
case R.id.checkBox2:
if (checked)
textview.setText("checkbox 2 is check by onclick from xml");
else
textview.setText("checkbox 2 is uncheck by onclick from xml");
break;
}
}
2.2. Or using an OnClickListener
checkbox1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
boolean checked = ((CheckBox) v).isChecked();
if (checked)
textview.setText("checkbox 1 is check by setOnClickListener");
else
textview.setText("checkbox 1 is uncheck by setOnClickListener");
}
});
checkbox2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
boolean checked = ((CheckBox) v).isChecked();
if (checked)
textview.setText("checkbox 2 is check by setOnClickListener");
else
textview.setText("checkbox 2 is uncheck by setOnClickListener");
}
});
3. How to get the setOnCheckedChangeListener for checkbox. This method is executed when there is any change in the state of the checkbox. You can change the state of checkbox from a button like:
button1.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// ==== change state of checkbox
checkbox1.setChecked(!checkbox1.isChecked());
}
});
And if you want to do something when state of checkbox is changed, you want to some code below:
checkbox1.setOnCheckedChangeListener(newCompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
// TODO Auto-generated method stub
if (buttonView.isChecked()) textview.setText("checkbox is check by button");
else textview.setText("checkbox is uncheck by button");
}
});
checkbox2.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
// TODO Auto-generated method stub
if (buttonView.isChecked()) textview.setText("checkbox 2 is check by button");
else textview.setText("checkbox 2 is uncheck by button");
}
});
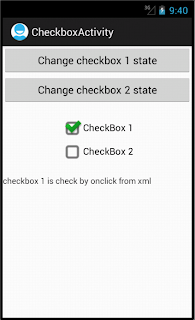
You can download all source codes from here
EmoticonEmoticon